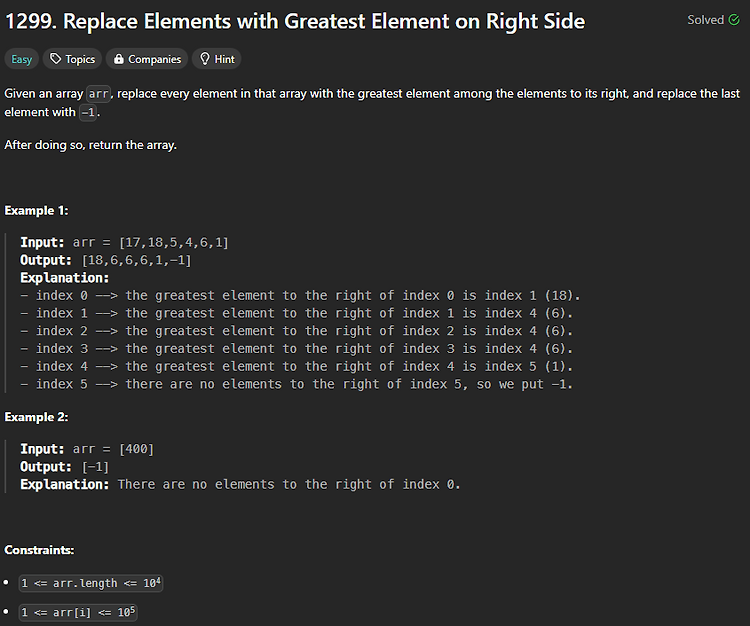
[LeetCode] 1299. Replace Elements with Greatest Element on Right Side, Easy
·
CodingTest/LeetCode
1. 문제배열 arr이 주어질 때, 0번 인덱스 부터 오른쪽에 위치한 값들 중 제일 높은 값으로 바꾸어 반환하라.마지막 요소는 -1로 채운다.2. 해결function replaceElements(arr: number[]): number[] { if(arr.length === 1) { // 요소가 1개만 있는 경우 -1로 채우고 반환. arr[arr.length-1] = -1; return arr; } let max = 0; // 최대값 저장. for(let i = arr.length - 1; i >= 0; i--) { // 뒤에서 부터 반복. if(i === arr.length - 1) { // 마지막 요소는 max를 업데이트하고 -1로 업..