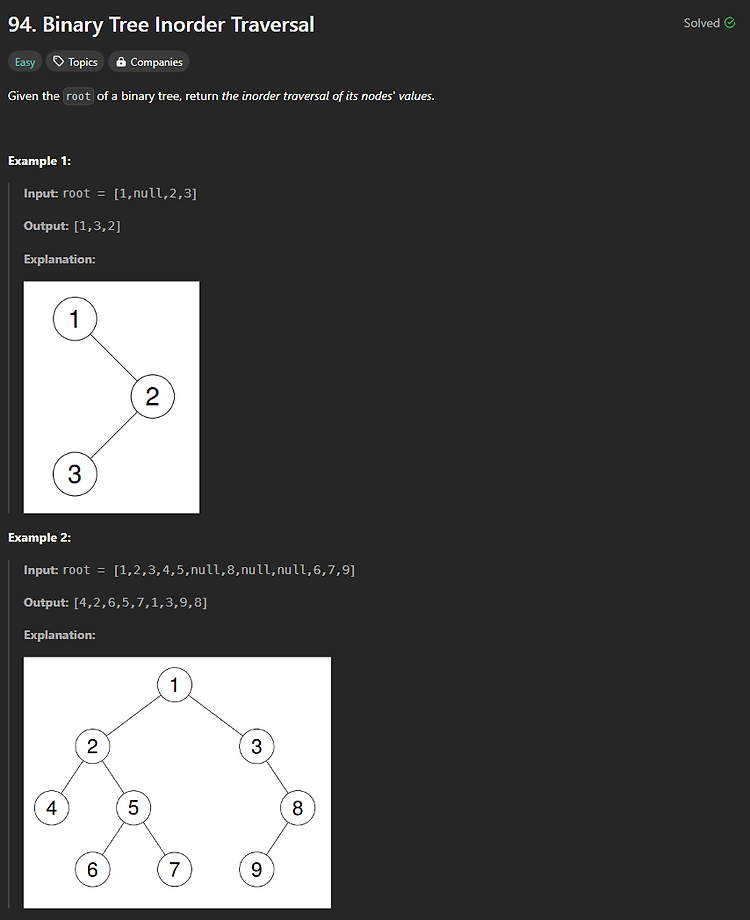
[LeetCode] 94. Binary Tree Inorder Traversal, Easy

·
CodingTest/LeetCode
1. 문제이진트리가 주어질 때, 중위 순회 결과를 반환하라.2. 해결/** * Definition for a binary tree node. * class TreeNode { * val: number * left: TreeNode | null * right: TreeNode | null * constructor(val?: number, left?: TreeNode | null, right?: TreeNode | null) { * this.val = (val===undefined ? 0 : val) * this.left = (left===undefined ? null : left) * this.right = (right===undefi..