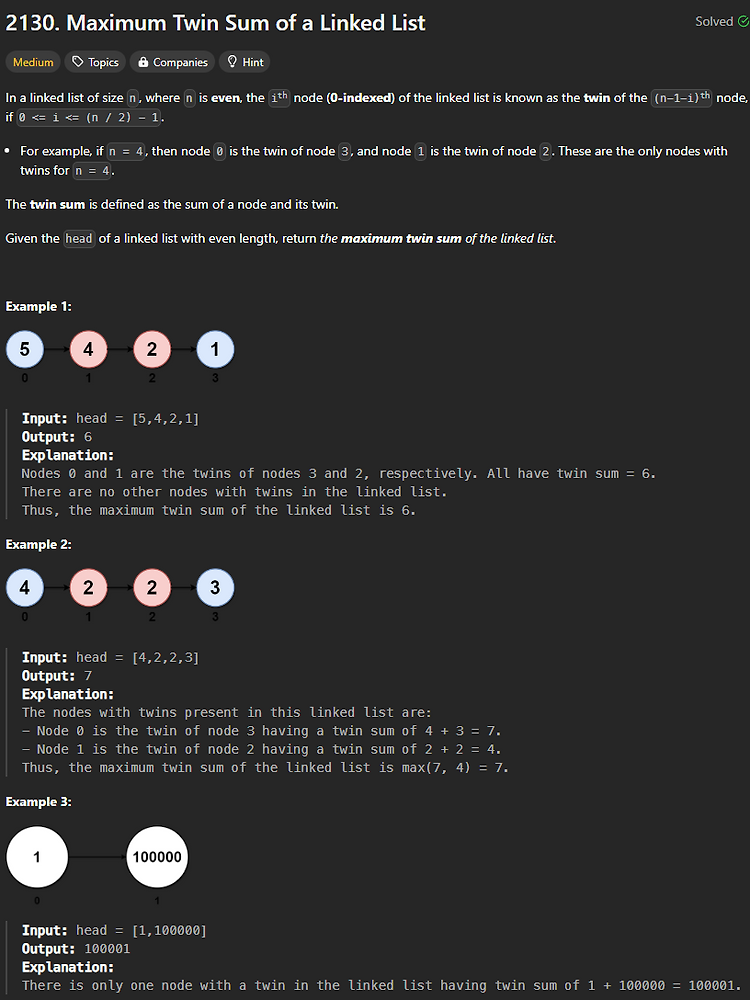
[LeetCode] 2130. Maximum Twin Sum of a Linked List, Medium
·
CodingTest/LeetCode
1. 문제짝수 개의 노드로 이루어진 연결 리스트가 주어질 때, 특정 노드의 인덱스와 쌍을 이루는 노드와의 최대 값을 구해라.쌍은 n - 1 - index 위치가 현재 노드의 쌍.2. 해결/** * Definition for singly-linked list. * class ListNode { * val: number * next: ListNode | null * constructor(val?: number, next?: ListNode | null) { * this.val = (val===undefined ? 0 : val) * this.next = (next===undefined ? null : next) * } * } */function rev..