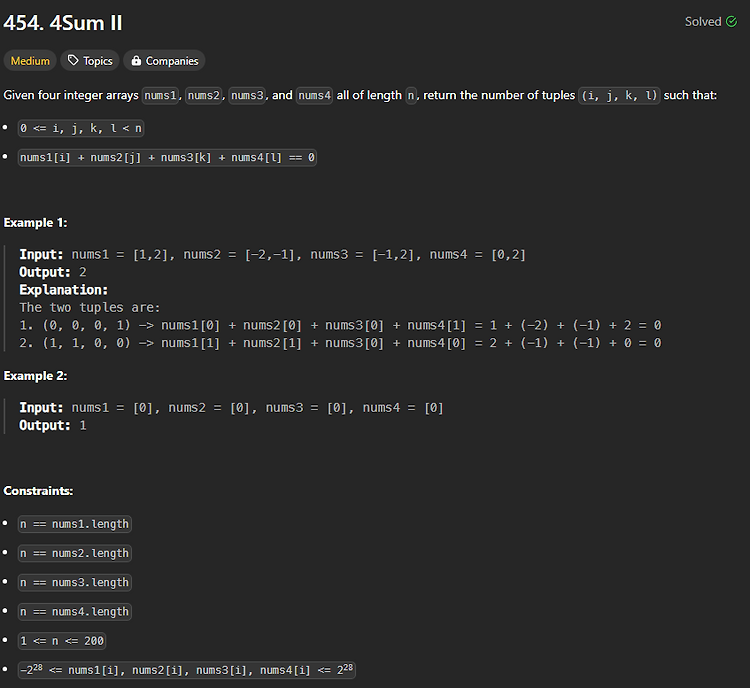
[LeetCode] 454. 4Sum II, Medium
·
CodingTest/LeetCode
1. 문제배열 4개가 주어질 때, 각 요소의 합이 0이되는 튜플의 수 를 구하라.2. 해결function fourSumCount(nums1: number[], nums2: number[], nums3: number[], nums4: number[]): number { const map = new Map(); const n = nums1.length; let ans = 0; for(let i =0; i4개의 배열이기 때문에 모든 경우의 수를 탐색하면 당연히 시간 초과가 날 것.근데 못풀었다.접근 방식이 안떠오른다 도저히.map을 이용해서 2개의 배열씩 등장할 수 있는 값과 등장 회수를 key-value로 관리다른 2개의 배열을 이용해 -를 붙여 해당 key가 있으면 등장 회수를 더해준다.