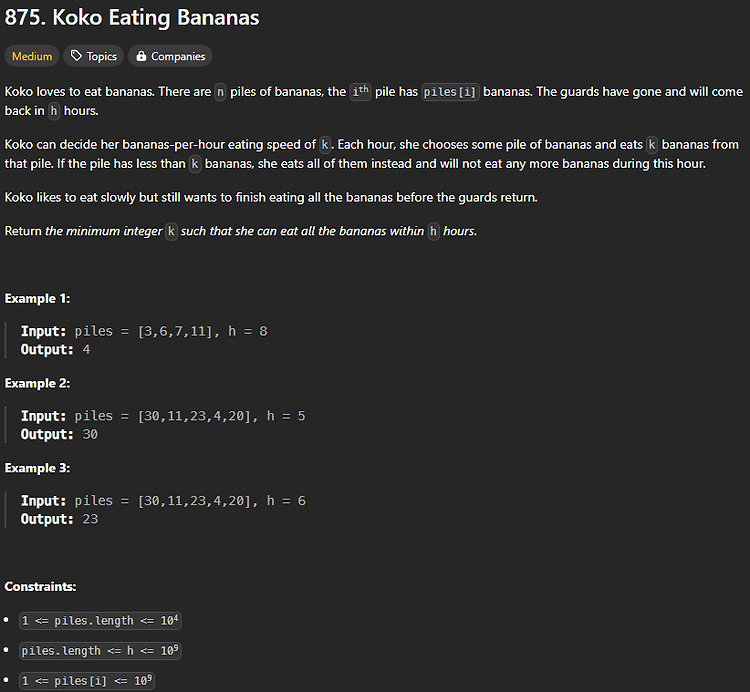
[LeetCode] 875. Koko Eating Bananas, Medium
·
CodingTest/LeetCode
1. 문제정수 배열 piles가 주어질 때, 1시간에 임의의 값 k만큼 각 요소를 삭제할 수 있다고 하자.정수 h시간 전에 모든 요소를 삭제할 수 있는 최소 k를 구하라.2. 해결function getTotalHours(piles: number[], k: number) : number { let total = 0; for(let pile of piles) { total += Math.ceil(pile / k); } return total;}function minEatingSpeed(piles: number[], h: number): number { let left = 1, right = Math.max(...piles); while(left 이진 탐색 문제에서..